Documentation
Activity Feed supports wide variety of options that allows you to customize the hell out of it.
Example
Activity Feed contains a few lightweight front-end modules, which are built as independent lego blocks. Together they form a robust component, which can be used for many different purposes. You can easily implement Facebook / Twitter-like posts, user timelines, activity streams and many other types of feeds.
-
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam nibh elit, gravida ac dignissim ut, malesuada ac risus. Nulla maximus odio ut mi dictum commodo.
<ul class="list-block">
<li class="list-block-item box">
<div class="media box-header">
<div class="media-left">
<div class="media-object">
<img src="http://i.pravatar.cc/50?img=14" alt="Name">
</div>
</div>
<div class="media-body">
<h3 class="media-title"><a href="#">Dave Lister</a></h3>
<div class="media-meta">
<small class="timestamp">27. 11. 2015, 15:00</small>
</div>
</div>
</div>
<div class="box-body">
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam nibh elit, gravida ac dignissim ut, malesuada ac risus. Nulla maximus odio ut mi dictum commodo. </p>
</div>
<div class="box-body">
<button class="icontype" data-toggle-class="is-active">
<span class="icontype-icon">
<svg viewBox="0 0 24 24" class="icontype-shape icontype-share">
<use class="shape-default" xlink:href="#shape-share"></use>
<use class="shape-active" xlink:href="#shape-share-inverse"></use>
</svg>
</span>
<span class="icontype-label">3</span>
</button>
<button class="icontype is-active" data-toggle-class="is-active">
<span class="icontype-icon">
<svg viewBox="0 0 24 24" class="icontype-shape icontype-heart">
<use class="shape-default" xlink:href="#shape-heart"></use>
<use class="shape-active" xlink:href="#shape-heart-inverse"></use>
</svg>
</span>
<span class="icontype-label">7</span>
</button>
<button class="icontype" data-toggle-class="is-active">
<span class="icontype-icon">
<svg viewBox="0 0 24 24" class="icontype-shape icontype-comment">
<use class="shape-default" xlink:href="#shape-comment"></use>
<use class="shape-active" xlink:href="#shape-comment-inverse"></use>
</svg>
</span>
<span class="icontype-label">23</span>
</button>
</div>
<div class="box-footer comment-list">
<div class="media comment">
<div class="media-left">
<div class="media-object">
<img src="http://i.pravatar.cc/32?img=18" alt="Name">
</div>
</div>
<div class="media-body">
<div class="comment-body">
<a class="comment-author" href="#">Dave Lister</a> <span>This is very nice feature</span></p>
</div>
<div class="comment-footer">
<abbr class="comment-date" title="21. duben 2017 v 16:28" data-utime="1492784927">3 min</abbr>
</div>
</div>
</div><!-- /comment -->
<div class="media comment">
<div class="media-left">
<div class="media-object">
<img src="http://i.pravatar.cc/32?img=18" alt="Name">
</div>
</div>
<div class="media-body">
<div class="comment-body">
<a class="comment-author" href="#">Dave Lister</a>
<span>Lorem ipsum dolor sit amet, consectetur adipiscing elit. In tortor ipsum, interdum sit amet augue ac, dictum faucibus nunc. Vestibulum non odio sit amet orci vehicula scelerisque quis vel ligula.</span> <span>Nam fermentum eu quam tincidunt imperdiet. Donec sapien nibh, porta ut hendrerit et, faucibus non tortor.</span>
</div>
<div class="comment-footer">
<abbr class="comment-date" title="21. duben 2017 v 16:28" data-utime="1492784927">3 min</abbr>
</div>
</div>
</div><!-- /comment -->
<div class="media comment-form is-collapsed">
<div class="media-left">
<div class="media-object">
<img src="http://i.pravatar.cc/32?img=18" alt="Name">
</div>
</div>
<div class="media-body">
<textarea class="form-control" placeholder="Leave a comment..." rows="1" cols="30"></textarea>
</div>
</div>
</div><!-- /comment-list -->
</li>
</ul>
Source structure
All source files are located in /src
folder. Each module has its own folder, so if you want to compile activity feed with your own variables, just include the whole folder into your project.
bootstrap-activity-feed/
└── src/javascripts
└── main.js - fallbacks for legacy browsers
└── src/stylesheets
├── index.less - Stylesheet Master File
└── modules
├── box
├── comments
├── icontype
├── image
├── list-block
└── media
└── util - Utility classes, common variables
Modules
Overview of all modules and their properties.
Module: List Block
Abstract container for the activity feed. General <ul>
list, which is striped of default styling. You can also use <div>
instead.
Name | Class | Usage |
---|---|---|
List Block | .list-block |
Container for list items. |
List Block Item | .list-block-item |
List item without specific styling |
<ul class="list-block">
<li class="list-block-item">List item 1</li>
<li class="list-block-item">List item 2</li>
<li class="list-block-item">List item 3</li>
</ul>
Module: Box
Generic box module extends block list and provides basic structure for the feed item.
Name | Class | Usage |
---|---|---|
Header | .box-header |
Header element, should contain title, primary action etc. |
Body | .box-body |
Main content of the box, can be used multiple times in single box element |
Footer | .box-footer |
Bottom element of the box, can be used fo secondary information, metadata etc. |
Actions | .box-actions |
Actions panel, absolutely positioned dropdown menu in top right corner |
<ul class="list-block">
<li class="list-block-item box">
<div class="box-header">Header</div>
<div class="box-body">Content</div>
<div class="box-footer">(optional) Footer</div>
<div class="box-actions">(optional) Actions</div>
</li>
</ul>
Module: Image
Common responsive image implementation. Images can be masked to fit specific aspect ratio.
Name | Class | Usage |
---|---|---|
Image | .image |
Wrapper for the image element <img> . Gives you many options how to manipulate the picture. |
Image Fit | .image-fit |
Add this class to fit image to its container and give it specific aspect ratio. Image Fit is by default adjusted to golden ratio. Available ratios, just add class to the wrapper element:
|
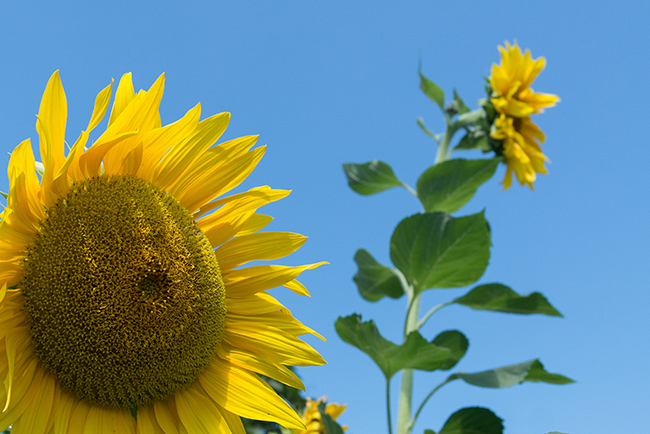
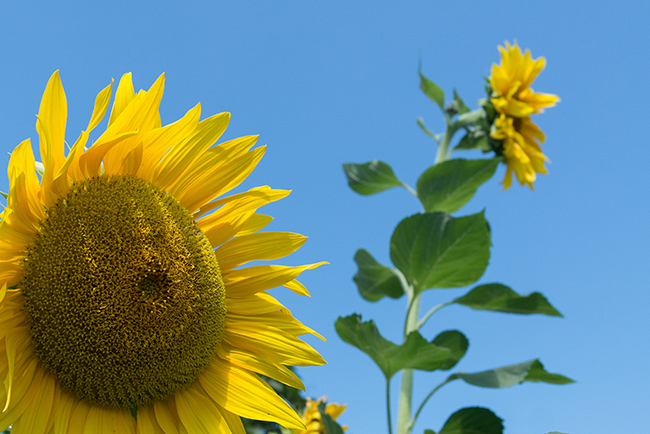
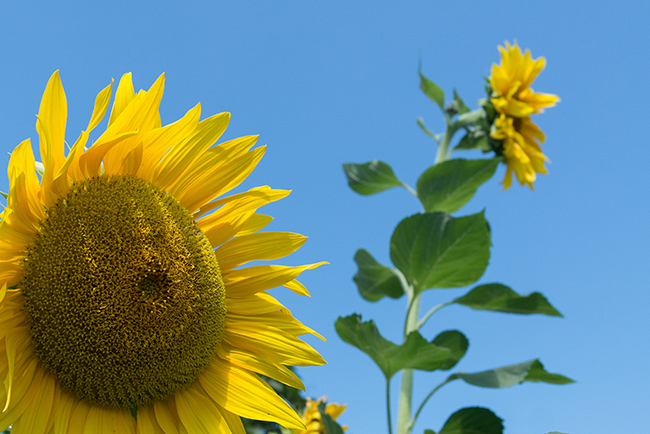
<div class="image image-fit ratio-square">
<img src="../assets/images/sunflower-650.jpg">
</div>
<div class="image image-fit ratio-3-2">
<img src="../assets/images/sunflower-650.jpg">
</div>
<div class="image image-fit ratio-16-9">
<img src="../assets/images/sunflower-650.jpg">
</div>
Module: Media Block
Extension for Bootstrap .media
block component. Adds some useful feature like exact media object width, custom paddings, media object with aspect ratio.
Name | Class | Usage |
---|---|---|
Media Block | .media-block |
Extension for Bootstrap
|
Media Fit | .media-fit |
Add class to the container of |
Media Title | .media-title |
Title of the media block. |
The Best Mentawai Islands Surf Video from my drone, Phyllis. June 2014, by Paul Borrud
Lorem ipsum dolor sit amet, consectetur adipiscing elit. In tortor ipsum, interdum sit amet augue ac, dictum faucibus nunc.
Module: Icontype
Icons with or without labels. If you use SVG symbols, you get predefined hover effects.
Name | Class | Usage |
---|---|---|
Icontype | .icontype |
Wrapper for the whole icon element, class can be applied to button , a or div
|
Icon | .icontype-icon |
Wrapper for icon symbol |
Label | .icontype-label |
Optional text label |
Shape | .icontype-shape |
Marks icon image, which can be SVG shape or bitmap image |
<button class="icontype">
<span class="icontype-icon">
<svg viewBox="0 0 24 24" class="icontype-shape icontype-heart">
<use class="shape-default" xlink:href="#shape-heart"></use>
<use class="shape-active" xlink:href="#shape-heart-inverse"></use>
</svg>
</span>
<span class="icontype-label">Like</span>
</button>
<button class="icontype is-active">
<span class="icontype-icon">
<svg viewBox="0 0 24 24" class="icontype-shape icontype-heart">
<use class="shape-default" xlink:href="#shape-heart"></use>
<use class="shape-active" xlink:href="#shape-heart-inverse"></use>
</svg>
</span>
<span class="icontype-label">You like this</span>
</button>
Module: Comments
Comments are structured media blocks, which extend basic Bootstrap .media
object. Comment media block provides common elements like avatar, comment message, reply form and metadata (author, post date, etc.).
Name | Class | Usage |
---|---|---|
Comment List | .comment-list |
Collection of comment elements |
Comment | .comment |
Extension for
|
Comment Form | .comment-form |
Container for a reply form |
<div class="comment-list">
<div class="media comment">
<div class="media-left">
<div class="media-object">
<img src="http://i.pravatar.cc/32" alt="Name">
</div>
</div>
<div class="media-body">
<div class="comment-body">
<a class="comment-author" href="#">Hadassah Hartman</a> Agupered nestu lipsa gerem
</div>
<div class="comment-footer">
<abbr class="comment-date" title="21. duben 2017 v 16:28" data-utime="1492784927">3 min</abbr>
</div>
</div>
</div><!-- /comment -->
<div class="media comment">
<div class="media-left">
<div class="media-object">
<img src="http://i.pravatar.cc/32" alt="Name">
</div>
</div>
<div class="media-body">
<div class="comment-body">
<a class="comment-author" href="#">Dave Lister</a>
<span>Lorem ipsum dolor sit amet, consectetur adipiscing elit. In tortor ipsum, interdum sit amet augue ac, dictum faucibus nunc. Vestibulum non odio sit amet orci vehicula scelerisque quis vel ligula.</span> <span>Nam fermentum eu quam tincidunt imperdiet. Donec sapien nibh, porta ut hendrerit et, faucibus non tortor.</span>
</div>
<div class="comment-footer">
<abbr class="comment-date" title="21. duben 2017 v 16:28" data-utime="1492784927">3 min</abbr>
</div>
</div>
</div><!-- /comment -->
<div class="media comment-form is-collapsed">
<div class="media-left">
<div class="media-object">
<img src="http://i.pravatar.cc/32" alt="Name">
</div>
</div>
<div class="media-body">
<textarea class="form-control" placeholder="Leave a comment..." rows="1" cols="30"></textarea>
</div>
</div><!-- /comment -->
</div><!-- /comment-list -->
We always strive to create better components. Feel free to send us any feedback, feature suggestions etc. → hello@skywalkapps.com.